When I try to use Hibernate search(5.9.1.Final) to sort the results, for example, name, the results was shown up with Uppercase first and Lowercase Second, like
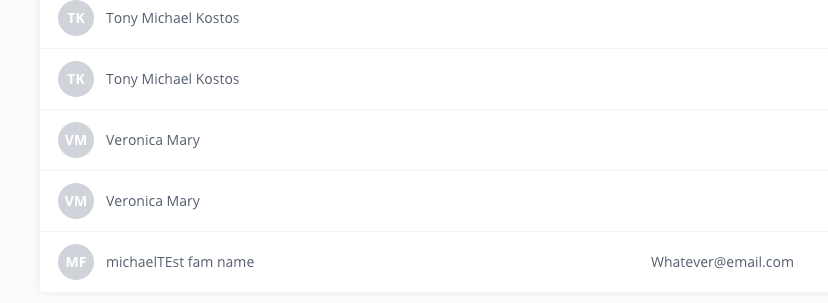
which does not make sense.
Code for entity annotation:
@Column(nullable = false, length = 50)
@Fields({
@Field(name = "firstName", index = Index.YES, analyze = Analyze.YES, analyzer = @Analyzer(definition = "nameAnalyzer")),
@Field(name = "dummyFieldToAddQueryAnalyzer", index = Index.YES, analyze = Analyze.YES, analyzer = @Analyzer(definition = "nameAnalyzer_query"), bridge = @FieldBridge(impl = DummyStringBridge.class)),
@Field(name = "firstName_sorted", analyze = Analyze.NO, store = Store.NO)
})
@SortableField(forField = "firstName_sorted")
private String firstName;
The sort method, I use query builder like
return qb.sort().byField(sortBy).desc().createSort();
Is there a way to change the sort order so that the lowercase letter has an equivalent sort order value than the uppercase letter.
You need to use a normalizer on your “firstName_sorted” field, and convert the name to lowercase in that normalizer.
Something like this:
// ==================================================
// HERE, add a normalizer definition, either like this or using programmatic definitions (see the links I give below)
// ==================================================
@NormalizerDef(
name = "sortNormalizer",
filters = {
@TokenFilterDef(factory = ASCIIFoldingFilterFactory.class), // To handle diacritics such as "é"
@TokenFilterDef(factory = LowerCaseFilterFactory.class)
}
)
public class MyEntity {
// ...
@Column(nullable = false, length = 50)
@Fields({
@Field(name = "firstName", index = Index.YES, analyze = Analyze.YES, analyzer = @Analyzer(definition = "nameAnalyzer")),
@Field(name = "dummyFieldToAddQueryAnalyzer", index = Index.YES, analyze = Analyze.YES, analyzer = @Analyzer(definition = "nameAnalyzer_query"), bridge = @FieldBridge(impl = DummyStringBridge.class)),
// ===================================================
// HERE, assign the normalizer and don't forget to enable analysis
// ===================================================
@Field(name = "firstName_sorted", analyze = Analyze.YES, normalizer = @Normalizer(name = "sortNormalizer"), store = Store.NO)
})
@SortableField(forField = "firstName_sorted")
private String firstName;
// ...
}
You can find all the necessary information in the documentation:
EDIT: You will need to reindex in order for these changes to be taken into account.
Thanks you for your help!!!
It works